-
Create a ChangeBug that will change to a truly random color every time it moves.
-
Create a trafficLightBug that will change colors to red, green and then blue then red again etc.
-
Write a TurningBug class that will move like a bug until it can't move. Then it will turn randomly to one of the 8 directions. Don't re-implement code that is already done.
-
Write a BackWard bug that acts like a bug except that he moves backwards.
-
Create a randomBug that will move at random to one of the empty positions around it. Have bug turn and face direction it is going to.
-
Write a salmonBug that moves like a randomBug. It will move 20 times and then it will turn around and follow back the path it took. If it can't move into a space because something else is there it will wait until the space is clear before it moves. Use an ArrayList (Stack for AB class) to store moves. After it follows its path back to its starting point it gives birth in all the adjacent spaces available and then it dies.
-
Create a darterBug that will try to move 2 spaces forward in direction it is going, otherwise tries to move one otherwise turns.
-
Create a slowBug that will move about 1 out of every 5 steps.
-
Create a flyingBug that will move randomly to any open spot on Grid. It must move every time.
-
Create a eastWest bug. It can only move east or west (start it facing East). It will keep going straight until it can't move, then it turns around. eastWest bugs are Blue.
-
Create a northSouth2 bug. It can only move north or south (start it facing North). It has a 50% chance of moving north and 50% chance of moving south. If it can't move it turns around.
-
Create a dynamicBug that will have a child on the order of about 1 out of 4 times and will die on the order of 1 out of 5 times. The bug will give birth in any one available location around it (at random).
-
Create a zigZagBug that will move to the left and the next time to the right (45 degrees). If it can't move then it should turn.
-
Create a flea. A flea will move like a normal bug but on every tenth move it will jump to an available random spot in the grid.
-
Create a slothBug that moves on every third step (not random).
-
Create a frogBug that will try to hop over an object that is adjacent to it if there is a place to land. If it can't hop it will move like a normal bug.
-
Create a rockEater (extend critter). It only eats rocks but moves like a critter.
-
Create a CheckerBug that extends the randomBug. It will hop over and kill any Bug that is in 1 of the spaces around it (horizontal, diagonal or vertical). It will only make the jump if there is a place to land. If it cannot hop over a Bug it will act like a normal randomBug.
-
Try to create a dodgingBug that will try to stay away from critters. The dodging bug can move in any direction like the randomBug but will try to move in the best direction to avoid being eaten. Set your bug in with 5 critters. How long did he last? Run it 1000 times. What is the average amount of times he lasted? Who can create the best dodging bug?
-
Create a crossingBug, It only travels North or South (start it out North). If it can't move forward it turns around. When it get to the top or the bottom of the Grid it will appear on the other side, if that space is available. If it is not available it will turn around.
-
Create a shootingbug that kills anything with x spaces of it in the direction it is facing. It moves like a random bug. When it is constructed it receives how far it can shoot. After you get him working release 10 Critters and 10 shootingBugs together in a 30 x 40 Grid. Members of which group will be the last one surving? The first time you run it construct shootingBugs being able to shoot 3 spaces. The next time increase it to 4, then 5 ... until you think he Critters and shootingBugs are on equal footing. On any given day members of one group or the other could survive.
-
Put 20 rocks into the Grid. Let the rock eater go until he has eaten all the rocks. How many moves did he have to make. (You can use System.out.println).
-
Once you get that working - put out 20 more rocks and let rockEater go. Run simulation 1000 times and have computer find average of how many moves rockEater had to make to clear the Grid of rocks. (Once grid is cleared you can add moves to a total, call a function to add rocks and let rock eater go again and let simulation continue.)
-
Create a breedingDyingBug that will die after it moves 10 times. It also has a 20% chance of having kids in the empty neighboring spots around it, each time it acts. breedingDyingBugs are green.
-
Create a RockingHorse that extends a Critter and will change any Flower around it to a Rock and then will move like a normal Critter.
|
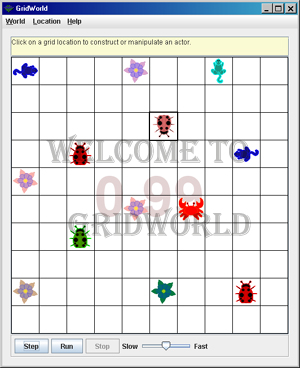
|